Virtual Try-On
If you're integrating the SDK for the first time, please use the artlabs Experience SDK. This SDK is deprecated and will be removed in the future.
Web
Install
You'll need to paste script code before closing </body>
tag of your web page
<script
type="module"
src="https://unpkg.com/meshkraft-vto/dist/index.min.js"
data-meshkraft-key="YOUR_API_KEY"
></script>
Make sure to replace YOUR_API_KEY
with your API key.
Basic Usage
After you include the script above, you need to call meshkraftVTO.init
function with your token and optional config object. Then, you're ready to present the experience by calling meshkraftVTO.startVTO
function.
meshkraftVTO.init({
token: 'YOUR_API_KEY',
// config is optional, see below for available options
config: { showAction: false, logoUrl: 'YOUR_LOGO_URL' },
});
meshkraftVTO.startVTO('YOUR_SKU');
That's it 🎉
Config
Possible values are;
CTA Banner Config
showAction
- Boolean. Should show action block or not. Default is false
actionButtonText
- String. Title for the button. Default is Add to Cart
actionButtonEventName
- String. Event name to listen on button click. Default is add-to-cart
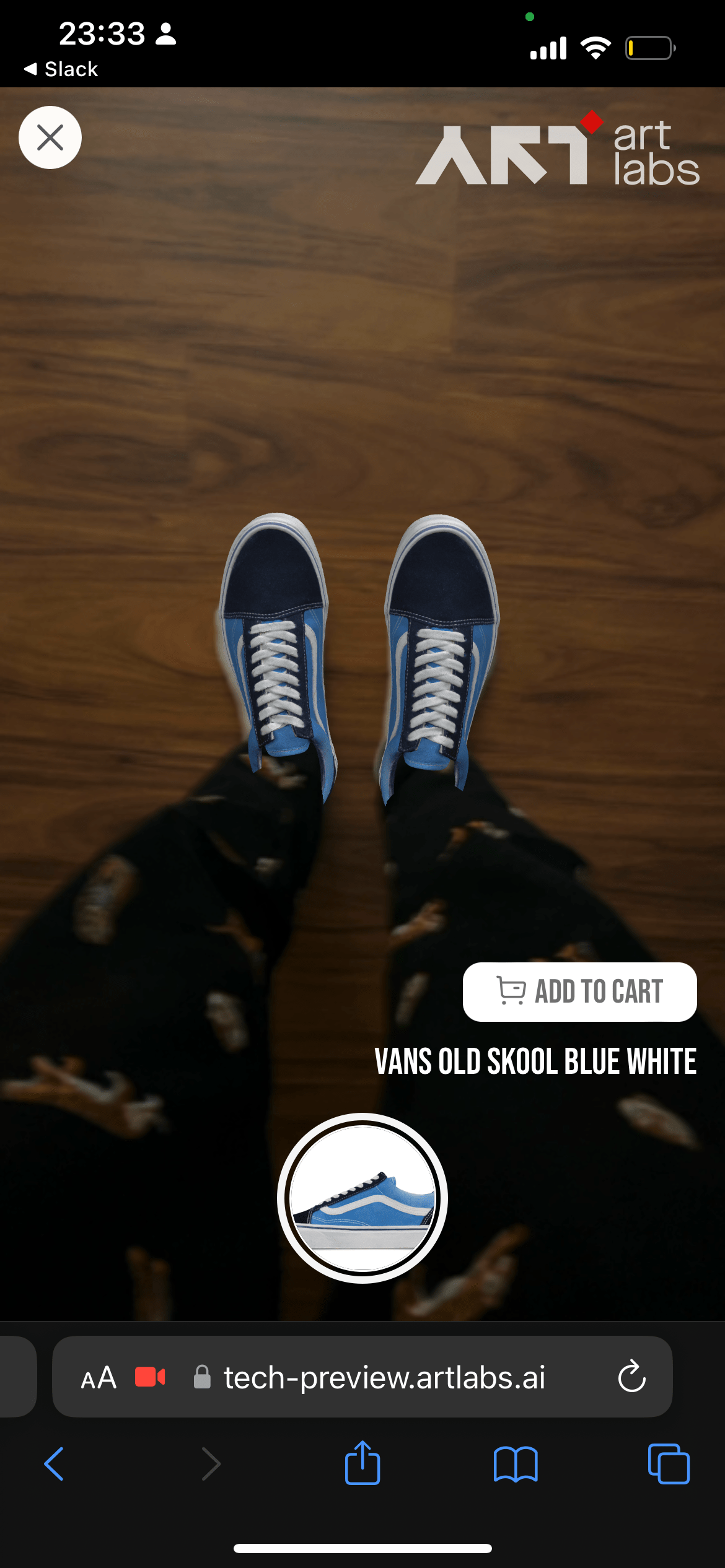
Branding Config
logoUrl
- String. Logo URL to use in upper-right corner. Default is null
fontFamily
- String. Font family name to use in SDK. Must be included in DOM. Default is Bebas Neue
qrPopupTitle
- String. Text to use in Desktop QR popup title. Default is Scan QR Code
qrPopupSubtitle
- String. Text to use in Desktop QR popup subtitle. Default is Point your mobile phone camera to the QR code below to experience Virtual Try-on
meshkraftVTO.init({
token: 'YOUR_API_KEY',
config: { logoUrl, fontFamily, showAction },
});
// or
meshkraftVTO.setConfig({ logoUrl, fontFamily, showAction });
Events
There are some events that can be listened to. You can register event listener by gettin element and invoking addEventListener
method.
Added to Cart
When user clicks the Add to Cart button in AR banner, this event will be fired with SKU.
// with functions
meshkraftVTO.addEventListener('add-to-cart', function (event) {
console.log('Size select requested with SKU: ' + e.detail.sku);
});
meshkraftVTO.addEventListener('close', function (event) {
console.log('Experience Closed on SKU: ' + e.detail.sku);
});
Error
When something goes wrong, this event will be fired with error message.
meshkraftVTO.addEventListener('vto-error', (e) => {
// VTO failed with reason
console.log(e.detail.message);
});
Features
SKU Variants
If your product has different colors or variants, implementing this function will allow you to have multiple variant representation on VTO experience.
To implement this feature ⬇️
The function should be called with comma seperated multiple SKU's:
meshkraftVTO.startVTO(“SKU-1,SKU-2,SKU-3,SKU-N”)
With the new feature, user will be able to see different colors on VTO experience with smooth transitions as the user navigates through your shoe's different color. 🎉
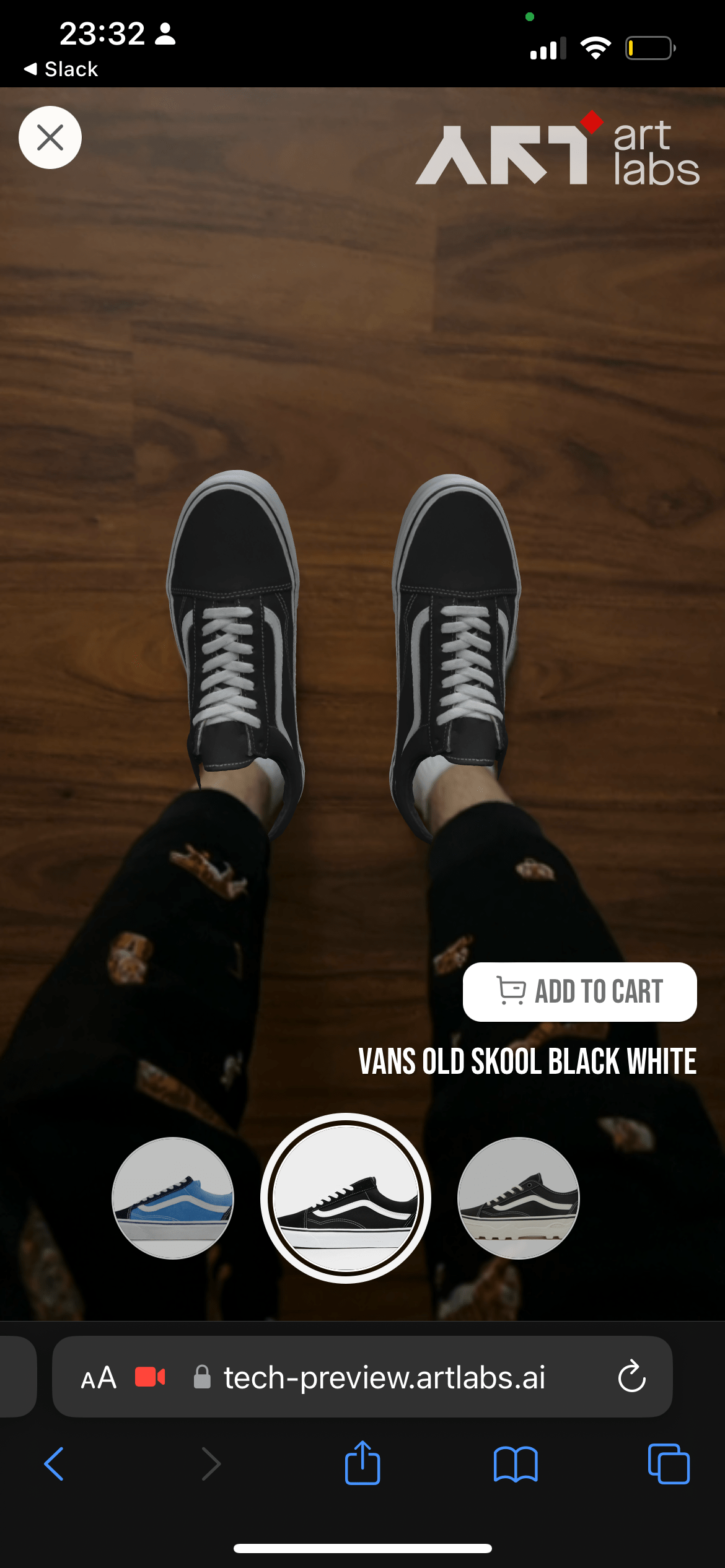
Full Example
<head>
<script
type="module"
src="https://unpkg.com/meshkraft-vto/dist/index.min.js"
data-meshkraft-key="YOUR_API_KEY"
></script>
</head>
<script>
// init with your token and config
meshkraftVTO.init({
token: 'YOUR_API_KEY',
config: { logoUrl, fontFamily, showAction },
});
// start experience by calling the function
meshkraftVTO.startVTO('Nike-Air-Max-90');
// add event listeners
meshkraftVTO.addEventListener('add-to-cart', function (event) {
console.log('Add to Cart requested with SKU: ' + e.detail.sku);
});
meshkraftVTO.addEventListener('close', function (event) {
console.log('Experience Closed on SKU: ' + e.detail.sku);
});
</script>
<!-- or just a HTML button -->
<body>
<button
id="meshkraft-vto-button"
data-meshkraft-vto-sku="Nike-Air-Max-90"
data-meshkraft-vto-config='{"showBanner": true, "buttonTitle":"Add To Cart", "buttonColor": "#4dad94", "textColor": "#fff"}'
></button>
</body>
Bundle Size
You'll be consuming minified and Gzipped or Brotli compressed versions, depending on the browser.
┌─────────────────────────────────────────────┐
│ │
│ meshkraft-vto.min.js │
│ Bundle Size: 19.17 KB │
│ Gzipped Size: 2.71 KB │
│ Brotli size: 157 bytes │
│ │
└─────────────────────────────────────────────┘
Initial bundle size is small, but the essential parts of the VTO will be downloaded after user clicks to the button. The total size will be about 2MB to 10MB, depending on the 3D model.
iOS
Please check this page for iOS specific instructions.
Android
Please check this page for iOS specific instructions.