3D Viewer
You have two options for web integrations. The first option provides both a 3D view and an AR button, while the second option goes directly into AR inside the phone camera.
For the time being, AR is only available on mobile devices because it requires a back camera to operate. In both options; when users try to open AR on the desktop, a popup window guides the user to their phone with a QR code.
1: Viewer & AR SDK
Install
The <artlabs-viewer>
web component can be used directly from either unpkg.com, npm or artlabs cdn
You'll need to paste script code before closing </body>
tag of your web page
<script
type="module"
src="https://unpkg.com/artlabs-viewer/dist/artlabs-viewer.min.js"
data-artlabs-key="YOUR_API_KEY"
></script>
Make sure to replace YOUR_API_KEY
with your API key.
Usage
After initialized, on a specific product page, call SDK with product identifier. (SKU)
<artlabs-viewer sku="YOUR_SKU" />
SDK will do the rest.
Options
Name | Default | Description |
---|---|---|
sku | required | SKU of your product. Identifier for 3D assets. |
width | 100% | CSS width of parent component. Accepts px, em, rem, vw. |
height | 300px | CSS height of parent component. Accepts px, vh, vw. |
auto-rotate | false | Should model rotate automatically. |
disable-interaction-prompt | false | Disables the interaction prompt that appears when the model is loaded. |
show-zoom-buttons | false | Shows zoom controls. |
reveal | auto | Determines when to load and reveal 3D model. Values; auto As soon as model loaded. interaction Reveals model after first user interactionmanual the model will remain hidden until dismissPoster() is called. |
config | null | Configuration object for additional settings. |
extra | null | Extra product information such as price, description to be displayed in AR banner. Required if show-banner enabled. |
show-banner | false | Should show a banner with Add to Cart button in iOS AR. |
banner-type | default | Type of iOS AR banner. Possible values are default , custom and apple-pay . Apple Pay includes a checkout with Apple Pay button. |
show-dimensions | false | Should show the dimensions of model in centimeters. |
custom-dimensions | null | Custom dimensions for the model, if different from default. |
show-wireframe | false | Displays the model in wireframe mode. |
viewer-style | null | Object to customize the viewer's style. |
disable-ar | false | Disables AR mode, hides View in AR button. |
disable-poster | false | Disables the poster image that appears before the model is loaded. |
disable-zoom | false | Disables zoom functionality. |
show-annotations | false | Enable model annotations. |
Methods
Name | Description |
---|---|
dismissPoster | Meant to use with reveal='manual' . Dismisses the poster image and loads 3D model |
Example;
const artlabsViewer = document.getElementById('artlabs-viewer');
artlabsViewer.dismissPoster();
Events
Some events can be listened to. You can register an event listener using addEventListener
method.
Added to Cart
When user clicks the Add to Cart button in AR banner, this event will be fired with SKU.
const artlabsViewer = document.querySelector('artlabs-viewer');
artlabsViewer.addEventListener('add-to-cart', (e) => {
// Add product to cart
console.log(e.detail.sku);
});
Full Example
<head>
<script
type="module"
src="https://unpkg.com/artlabs-viewer/dist/artlabs-viewer.min.js"
data-artlabs-key="YOUR_API_KEY"
></script>
</head>
<body>
<artlabs-viewer
sku="Nike-Air-Max-90"
height="500px"
show-banner
banner-type="default"
extra='{"price": "$500", "description":"White, Essential"}'
></artlabs-viewer>
</body>
<script>
const artlabsElement = document.querySelector('artlabs-viewer');
artlabsElement.addEventListener('add-to-cart', (e) => {
console.log('Product added to cart :: ', e.detail.sku);
});
</script>
UX Recommendations
When integrating 3D visualization into your product pages, you have two primary implementation strategies:
-
First Page Load Integration: Include the 3D viewer directly in your initial page load for an immediate immersive experience. This works well for products where 3D visualization is a key selling point.
-
Manual 2D/3D Switch: Implement a toggle between traditional 2D images and 3D visualization, giving users control over their viewing experience. This approach is ideal when you want to maintain a familiar interface while offering enhanced 3D capabilities.
Here's an example of a clean product page example with a "Show in 3D" button:
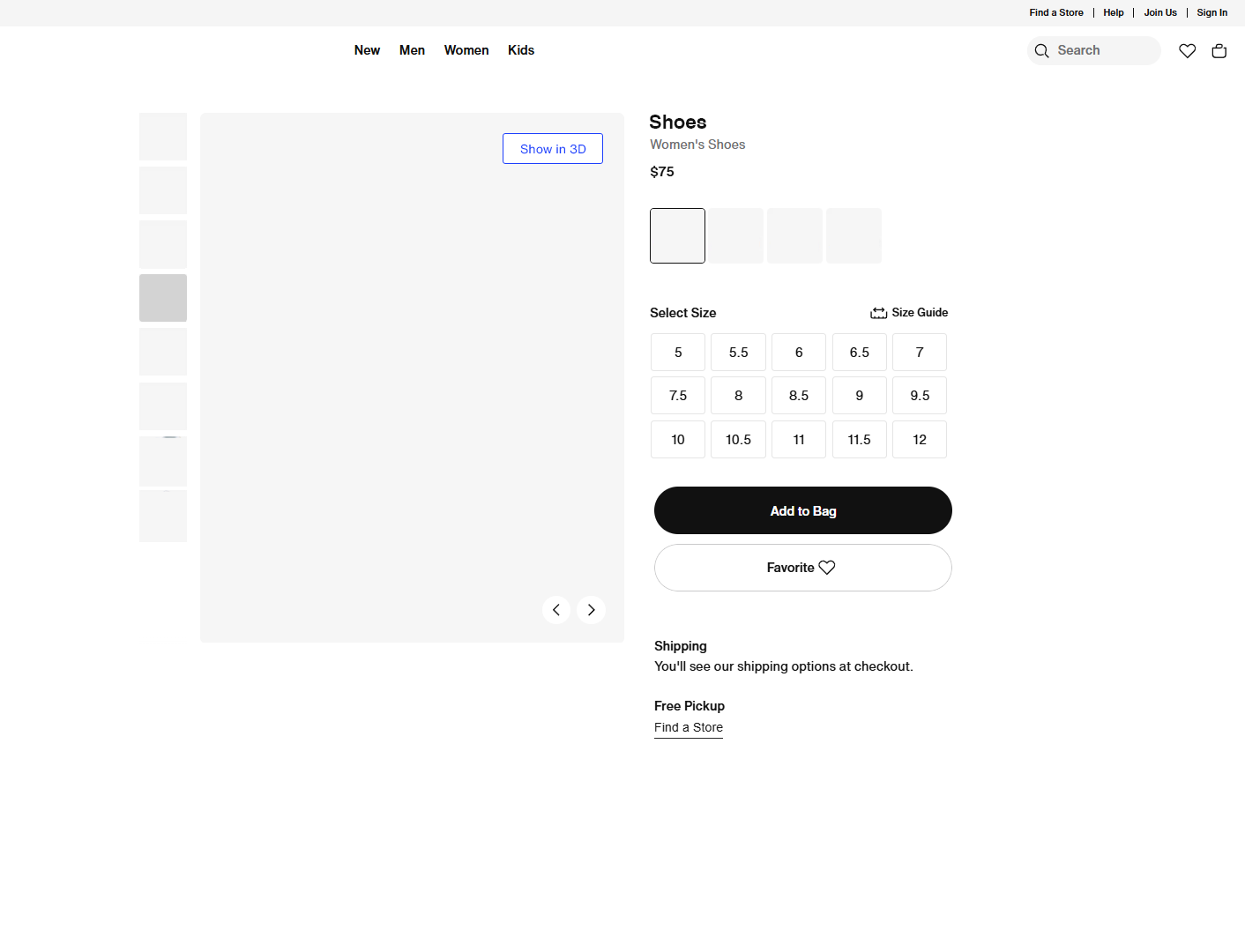
Bundle Size
artlabs-viewer loads progressively. Initial load is very small, around 20kb. As the browser requires, it will load additional assets.